Introducing Hookdeck Vercel Middleware (Deprecated)
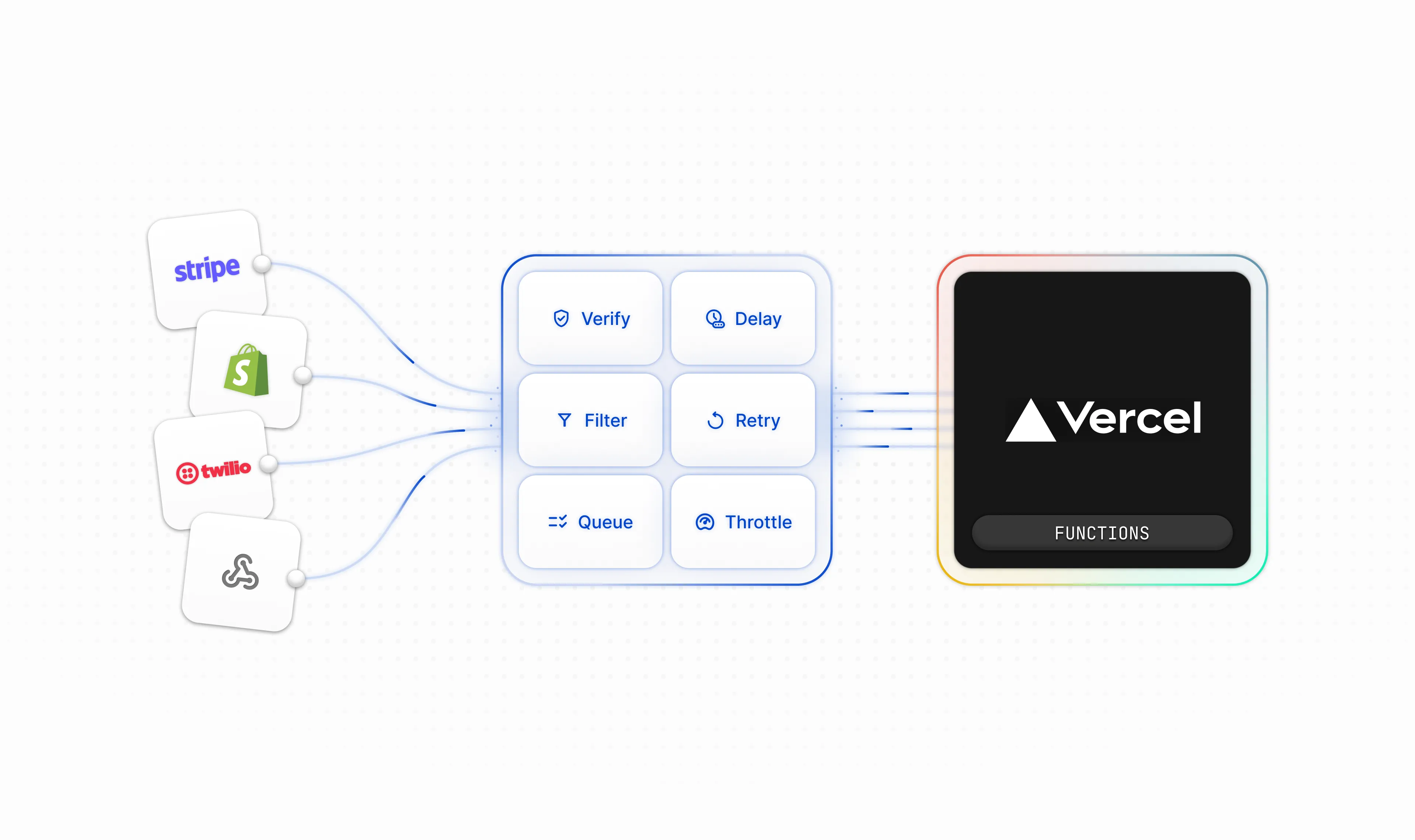
The Hookdeck Vercel Middleware has been Deprecated. Get in touch if you have any questions.
Every month, we deliver hundreds of thousands of asynchronous events to Vercel application endpoints, so we want to make debugging and building with webhooks and other asynchronous messaging use cases on Vercel even easier.
So, today, we're pleased to announce the Hookdeck Vercel Middleware, which enables you to turn any of your endpoints on Vercel, irrespective of framework, into asynchronous endpoints with support for queuing, throttling, retry logic, delays, and verification.
In the following video, Alex walks through using the Hookdeck Vercel Middleware in a Next.js application:
You can find the Hookdeck Vercel Middleware on GitHub. The repository README also includes all middleware configuration options, including retry logic, delays, filtering, throttling, verification, and more.
If you'd prefer to begin with a pre-configured example, there's a Next.js example on GitHub.
Get Started with the Hookdeck Vercel Middleware
To get started with the Hookdeck Vercel Middleware, install the Hookdeck Vercel Middleware package on the project that you deploy to Vercel:
npm i @hookdeck/vercel
The installation process creates one if you don't already have a middleware.ts
(or middleware.js
) file. If you already have a middleware file, update it to use the Hookdeck Vercel Middleware and update the matcher
value to match the route you want to add queueing, throttling, etc. to.
In the example below, we're adding the middleware to a route that matches /api/webhooks/stripe
to handle your valuable Stripe webhooks:
// add Hookdeck imports
import { withHookdeck } from '@hookdeck/vercel';
import hookdeckConfig from './hookdeck.config';
export const config = {
matcher: '/api/webhooks/stripe',
};
// the middleware is not exported anymore
function middleware(request: Request) {
// ... your middleware logic
// return `NextResponse.next()` or `next()` to manage the request with Hookdeck
}
// wrap the middleware with Hookdeck wrapper
export default withHookdeck(hookdeckConfig, middleware);
As part of the package install, a hookdeck.config.js
will be added to your application codebase. Update the match
value to match the route in your middleware file. For example, for the above Stripe webhooks example, you'd update the match
value to /api/webhooks/stripe
:
const { RetryStrategy, DestinationRateLimitPeriod } = require('@hookdeck/sdk/api');
/** @type {import("@hookdeck/vercel").HookdeckConfig} */
const hookdeckConfig = {
match: {
'/api/webhooks/stripe': {
retry: {
strategy: RetryStrategy.Linear,
count: 5,
interval: 1 * 60 * 1000, // in milliseconds
},
rate: {
limit: 10,
period: DestinationRateLimitPeriod.Second,
},
},
},
};
module.exports = hookdeckConfig;
Next, create a Hookdeck account, head to your project secrets set the following Vercel environment variables in your project:
HOOKDECK_API_KEY
with your Hookdeck API KeyHOOKDECK_SIGNING_SECRET
with your Hookdeck Signing Secret
Finally, deploy your Vercel project, and you're all set. The next time a request is made that matches the configured middleware routes, it will be managed by the Hookdeck Vercel Middleware.
Note: The Hookdeck Vercel Middleware is currently disabled in development environments (detected by
VERCEL_ENV
andNODE_ENV
environment variables). We'll be adding support for this soon.
Learn more
You can find more information in the following resources: